Requirements
- Arduino nano
- ACS712 current sensor
- ESP8266 wifi module
- Backend (PHP server in our case)
- Webserver to serve static html files
- Sequel database tot store the records when someone rang the bell
Hello reader, this post is about a smart doorbell.
The concept was to create a smart doorbell. Wich wasn’t expensive and hard to install.
What was achieved was something smart and easy to install on your existing electric installation wich sends you a message on your smartphone when someone rings your doorbell.
To push notifications to the smartphone of the user the firebase cloud messaging service was used.
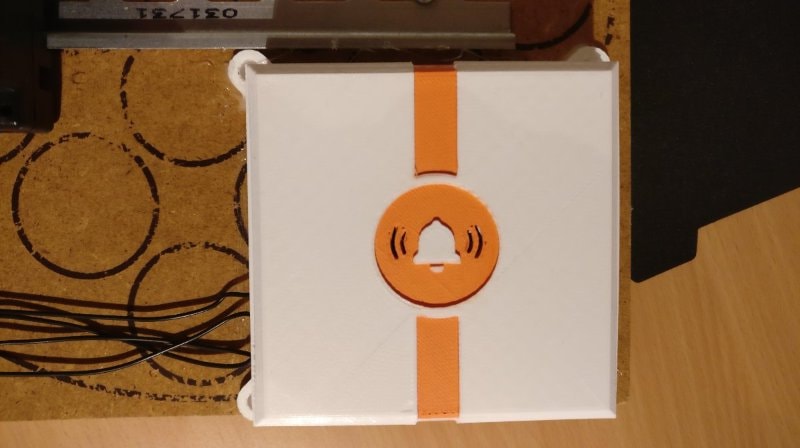
Arduino Code to measure current
/*
Measuring AC Current Using ACS712
*/
const int sensorIn = A0;
int mVperAmp = 66;
double Voltage = 0;
double VRMS = 0;
double AmpsRMS = 0;
int count = 0;
void setup()
{
Serial.begin(57600);
}
void loop()
{
Voltage = getVPP();
VRMS = (Voltage / 2.0) * 0.707;
AmpsRMS = (VRMS * 1000) / mVperAmp;
}
float getVPP()
{
float result;
int readValue; //value read from the sensor
int maxValue = 0; // store max value here
int minValue = 1024; // store min value here
uint32_t start_time = millis();
while ((millis() - start_time) < 1000) //sample for 1 Sec
{
readValue = analogRead(sensorIn);
// see if you have a new maxValue
if (readValue > maxValue)
{
/*record the maximum sensor value*/
maxValue = readValue;
}
if (readValue < minValue)
{
/*record the maximum sensor value*/
minValue = readValue;
}
}
// Subtract min from max
if (count != 0)
{
count--;
}
result = ((maxValue - minValue) * 5.0) / 1024.0;
//Serial.println(result);
if (result > 0.050 && count == 0)
{
count = 5;
Serial.print("P");
//delay(5000);
}
return result;
}
Cool bro! 😁